Q:
Is an Emoji (or Unicode Character) available on a given device?
A:
Maybe. ← Compare against this character! 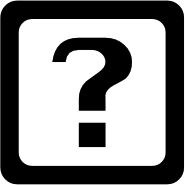
This article goes into details on how to compare the emoji or Unicode rendition against a known, invalid image.
This approach is very similar to the algorithm I implemented back in 1993, which was the object of a patent application.
It is also the response proposed to Stack Overflow question #41318999, «Is there a way to know if an Emoji is supported in iOS?»
-
SO-41318999
-
SO-9352753
-
SO-31363211
Method: Compare character images
- Convert the character into a Portable Network Graphic image, lossless
- Extract the png data
- Compare the data against a known invalid image
- If there is a match, then the character is invalid
This approach leverages on the fact that the OS uses the same default character for unknown unicode character.
Step 1: character to png
func png(ofSize fontSize: CGFloat) -> Data? { let attributes = [NSAttributedStringKey.font: UIFont.systemFont(ofSize: fontSize)] let charStr = "\(self)" as NSString let size = charStr.size(withAttributes: attributes) UIGraphicsBeginImageContext(size) charStr.draw(at: CGPoint(x: 0,y :0), withAttributes: attributes) var png:Data? = nil if let charImage = UIGraphicsGetImageFromCurrentImageContext() { png = UIImagePNGRepresentation(charImage) } UIGraphicsEndImageContext() return png }
Step 2: Compare against a known invalid character
func unicodeAvailable() -> Bool { if let refUnicodePng = Character.refUnicodePng, let myPng = self.png(ofSize: Character.refUnicodeSize) { return refUnicodePng != myPng } return false }
► Find the complete project on GitHub (Swift + Xcode) and run your own Unit Tests to experiment.